Assembler, Linker, and Simulator
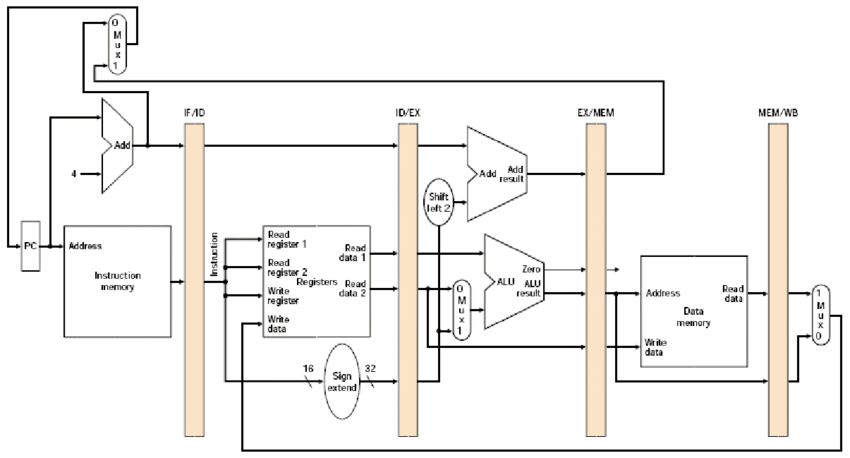
A system for visualizing a single-cpu executing assembly code
Tags:
Description
This project is a set of 4 sub-projects done for my EECS 370: Intro to Computer Organization class in the Winter 2022 semester. Each sub project involved building some part of the pipeline to run assembly code. The entire project was coded in C.
Sub Projects:
Project 1: Assembler and Single Cycle Simulator This project involved writing a program that received assembly code as the input and outputted machine code (binary integers) as output. These machine code files could be fed into a simulator, the creation of which was the second part of the project. The simulator took in a machine code file and simulated the state of a single cycle processor running the machine code program. Finally, the third part of the project was the writing of an assembly language program that multiplied two numbers.
Project 2: Object File (ELF) Assembler and Linker This project involved modifying the assembler from Project 1 to produce object files in a linux ELF-like format. These files could then be linked together using a Linker, the creation of which was the second part of the project. This allowed for running multi-file programs with global labels that could reference the other files.
Project 3: Pipelined Simulator This project involved modifying the simulator from Project 1 to run programs in a pipelined manner, executing closer to a single instruction per ‘processor’ cycle.
Project 4: Cache simulator This project involved modifying the pipelined simulator from Project 4 to add a cache for faster memory performance.
Skills Learned:
- Assembly language: the assembly language used in these projects is named LC2K, and features a simplified instruction that is based on ARM
- C Programming Language: Before this project, I had never used C. Making the step back down to the older C (which lacked features I had come to love in C++ such as classes and the STL) was rough, but I learned a lot and was able to transfer over many of my C++ skills.
- Bit manipulation: This project featured extensive use of bit masking and manipulation in C, something that I had never used in C++ coding
- Optimization: learning how to optimize space and memory was a key part of this project. I learned of things such as struct padding and how the stack works, which is important general knowledge for programmers, especially in C.
- Linux ELF: knowledge of the Linux Executable and Linkable format was an important factor in learning how something like a compiler works, and is essential knowledge for someone working in the Linux space
- Processor - Learning how single cycle and multicycle processors work is important for developers, as it allows them to program in a way that is conscious of the hardware they are operating on; for example, learning how memory is cached can be used to write programs that use more localized memory accesses to run faster.